Media Library
The Media Library is the Strapi feature that displays all assets uploaded in the Strapi application and allows users to manage them.
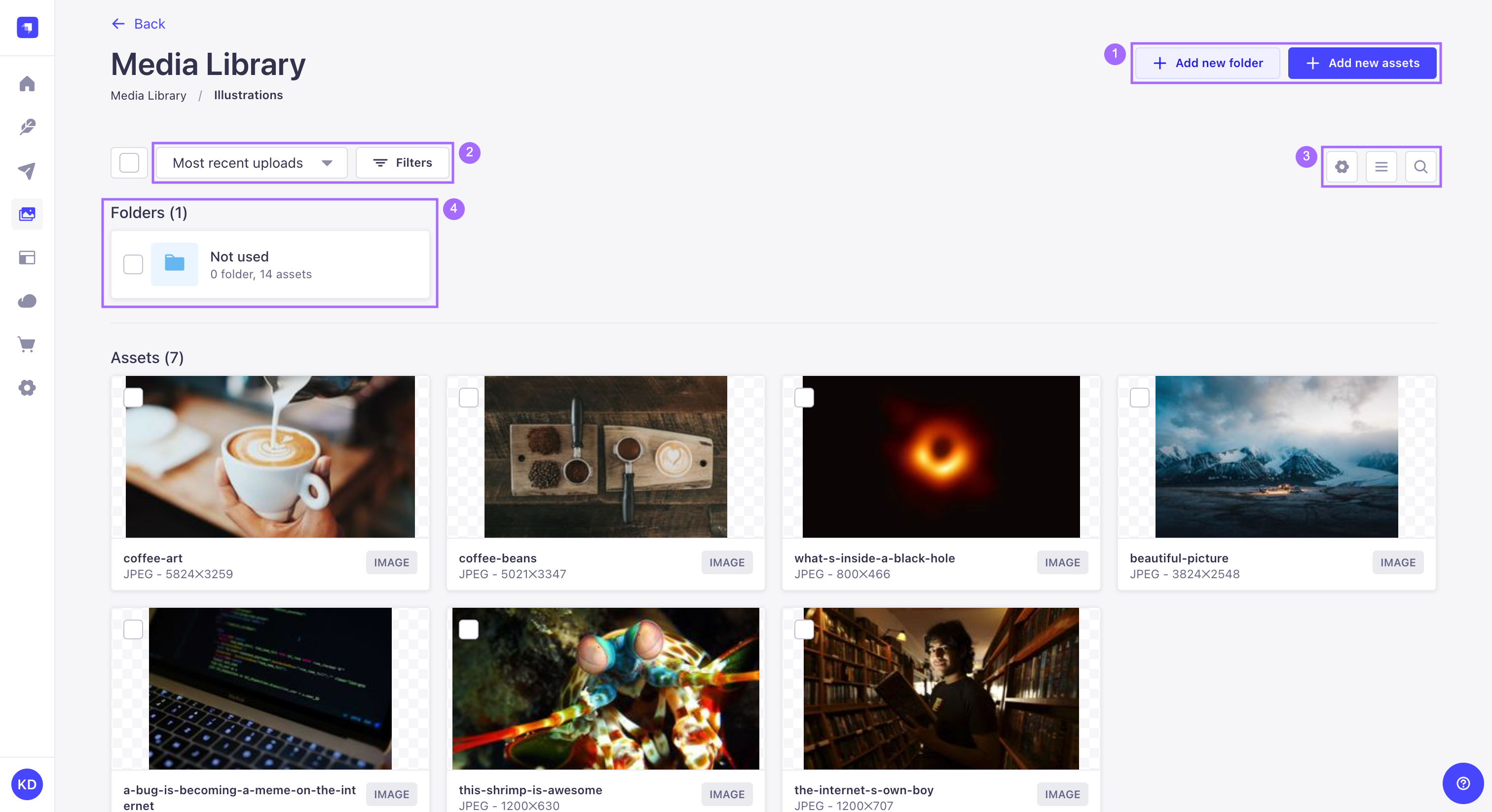
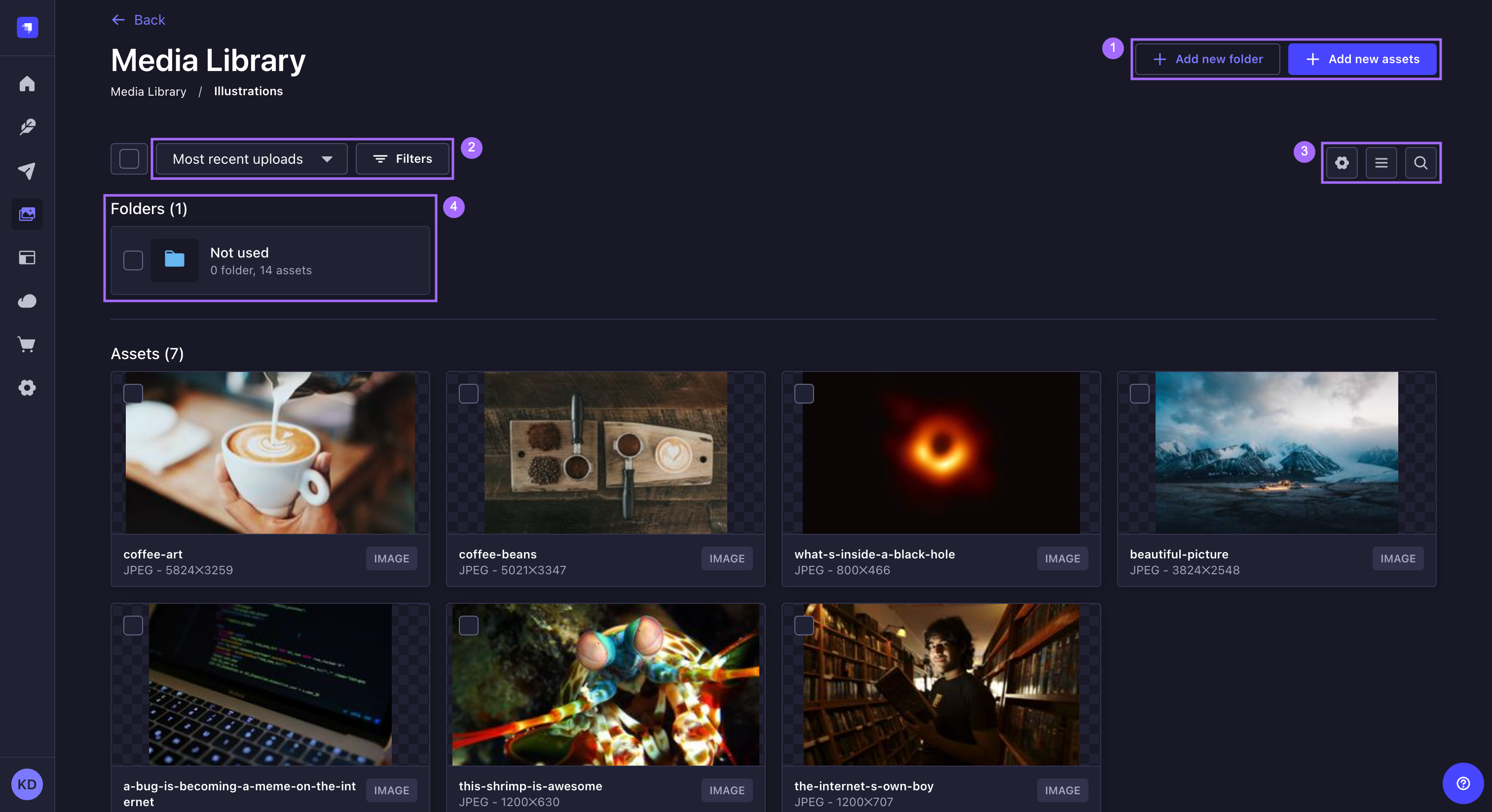
Plan: Media Library is a free feature.
Role & permission: Minimum "Access the Media Library" permission in Roles > Plugins - Upload.
Activation: Available and activated by default.
Environment: Available in both Development & Production environment.
Configuration
Some configuration options for the Media Library are available in the admin panel, and some are handled via your Strapi project's code.
Admin panel settings
The Media Library settings allow controlling the format, file size, and orientation of uploaded assets.
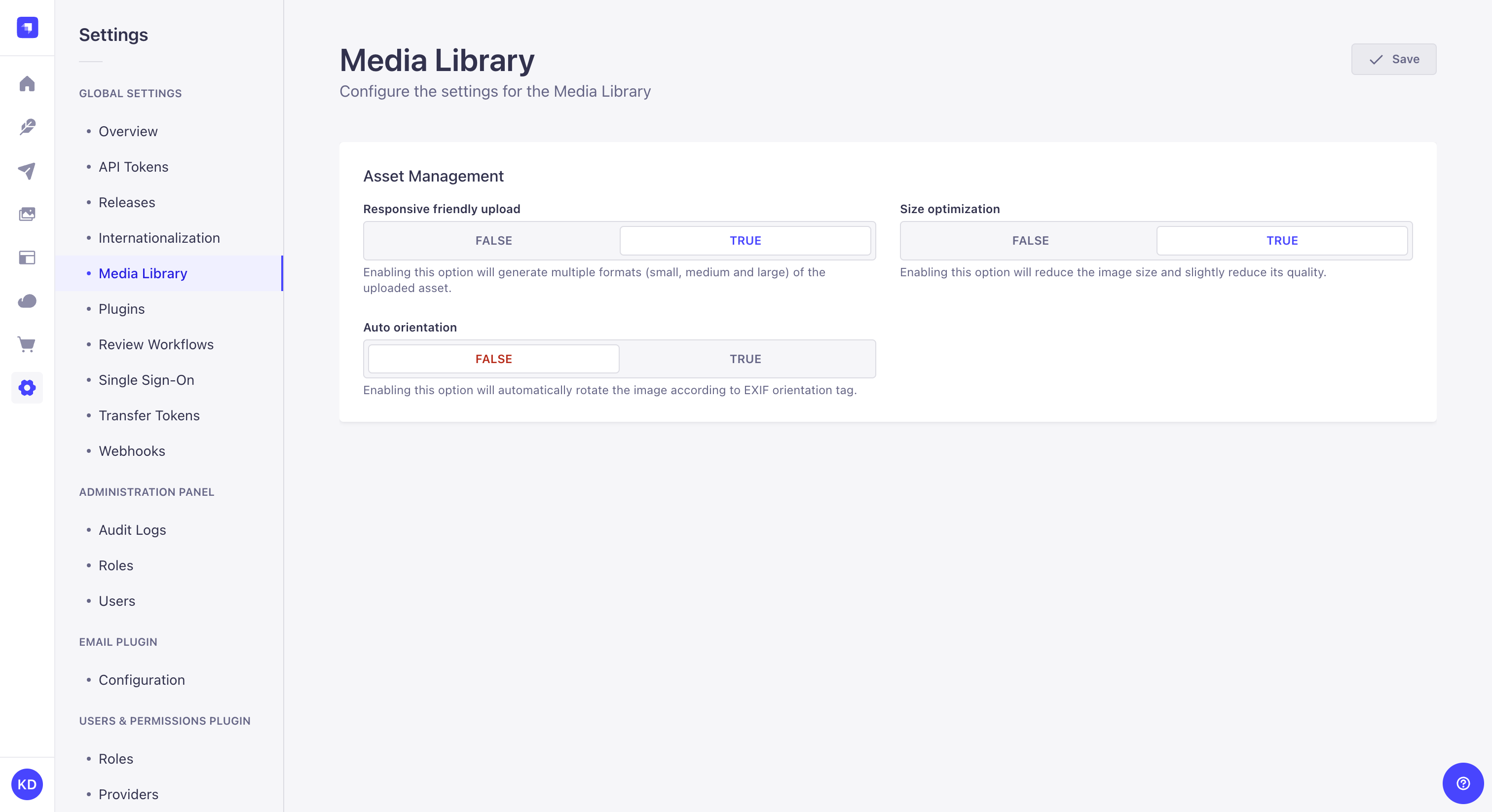
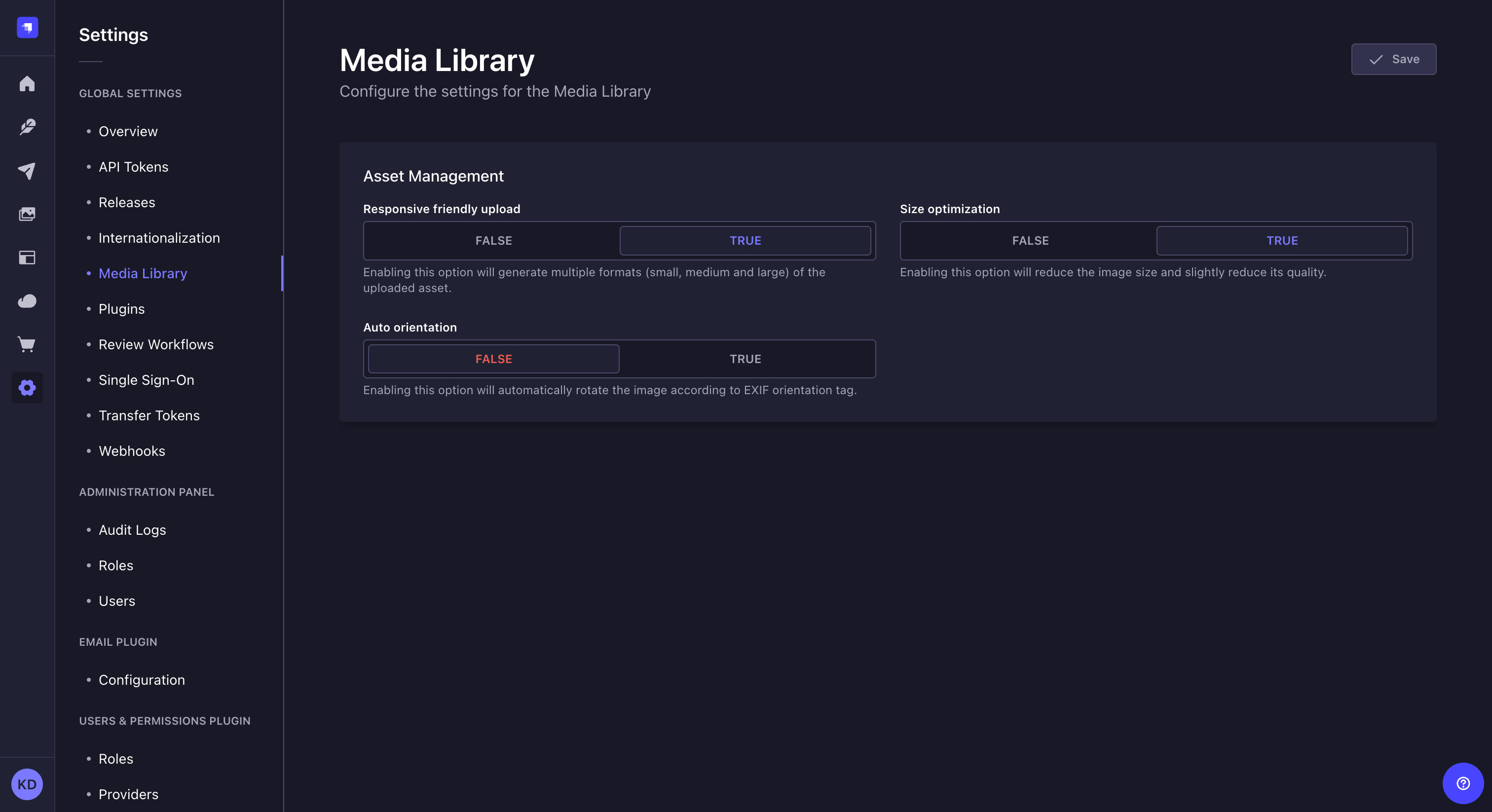
-
Go to
Settings > Global Settings > Media Library.
-
Define your chosen new settings:
Setting name Instructions Default value Responsive friendly upload Enabling this option will generate multiple formats (small, medium and large) of the uploaded asset.
Default sizes for each format can be configured through the code.True Size optimization Enabling this option will reduce the image size and slightly reduce its quality. True Auto orientation Enabling this option will automatically rotate the image according to EXIF orientation tag. False -
Click on the Save button.
Code-based configuration
The Media Library is powered in the backend server by the Upload package, which can be configured and extended through providers.
Additional providers
By default Strapi provides a provider that uploads files to a local public/uploads/
directory in your Strapi project. Additional providers are available should you want to upload your files to another location.
The providers maintained by Strapi are the following. Clicking on a card will redirect you to their Strapi Marketplace page:
Amazon S3
Official provider for file uploads to Amazon S3.
Cloudinary
Official provider for media management with Cloudinary.
Local
Default provider for storing files locally on the server.
Code-based configuration instructions on the present page detail options for the default upload provider. If using another provider, please refer to the available configuration parameters in that provider's documentation.
Available options
When using the default upload provider, the following specific configuration options can be declared in an upload.config
object within the config/plugins
file. All parameters are optional:
Parameter | Description | Type | Default |
---|---|---|---|
providerOptions.localServer | Options that will be passed to koa-static upon which the Upload server is build (see local server configuration) | Object | - |
sizeLimit | Maximum file size in bytes (see max file size) | Integer | 209715200 (200 MB in bytes, i.e., 200 x 1024 x 1024 bytes) |
breakpoints | Allows to override the breakpoints sizes at which responsive images are generated when the "Responsive friendly upload" option is set to true (see responsive images) | Object | { large: 1000, medium: 750, small: 500 } |
The Upload request timeout is defined in the server options, not in the Upload plugin options, as it's not specific to the Upload plugin but is applied to the whole Strapi server instance (see upload request timeout).
Example custom configuration
The following is an example of a custom configuration for the Upload plugin when using the default upload provider:
- JavaScript
- TypeScript
module.exports = ({ env })=>({
upload: {
config: {
providerOptions: {
localServer: {
maxage: 300000
},
},
sizeLimit: 250 * 1024 * 1024, // 256mb in bytes
breakpoints: {
xlarge: 1920,
large: 1000,
medium: 750,
small: 500,
xsmall: 64
},
},
},
});
export default () => ({
upload: {
config: {
providerOptions: {
localServer: {
maxage: 300000
},
},
sizeLimit: 250 * 1024 * 1024, // 256mb in bytes
breakpoints: {
xlarge: 1920,
large: 1000,
medium: 750,
small: 500,
xsmall: 64
},
},
},
})
Local server
By default Strapi accepts localServer
configurations for locally uploaded files. These will be passed as the options for koa-static.
You can provide them by creating or editing the /config/plugins
file. The following example sets the max-age
header:
- JavaScript
- TypeScript
module.exports = ({ env })=>({
upload: {
config: {
providerOptions: {
localServer: {
maxage: 300000
},
},
},
},
});
export default ({ env }) => ({
upload: {
config: {
providerOptions: {
localServer: {
maxage: 300000
},
},
},
},
});
Max file size
The Strapi middleware in charge of parsing requests needs to be configured to support file sizes larger than the default of 200MB. This must be done in addition to provider options passed to the Upload package for sizeLimit
.
You may also need to adjust any upstream proxies, load balancers, or firewalls to allow for larger file sizes. For instance, Nginx has a configuration setting called client_max_body_size
that must be adjusted, since its default is only 1mb.
The middleware used by the Upload package is the body
middleware. You can pass configuration to the middleware directly by setting it in the /config/middlewares
file:
- JavaScript
- TypeScript
module.exports = [
// ...
{
name: "strapi::body",
config: {
formLimit: "256mb", // modify form body
jsonLimit: "256mb", // modify JSON body
textLimit: "256mb", // modify text body
formidable: {
maxFileSize: 250 * 1024 * 1024, // multipart data, modify here limit of uploaded file size
},
},
},
// ...
];
export default [
// ...
{
name: "strapi::body",
config: {
formLimit: "256mb", // modify form body
jsonLimit: "256mb", // modify JSON body
textLimit: "256mb", // modify text body
formidable: {
maxFileSize: 250 * 1024 * 1024, // multipart data, modify here limit of uploaded file size
},
},
},
// ...
];
In addition to the middleware configuration, you can pass the sizeLimit
, which is an integer in bytes, in the /config/plugins file:
- JavaScript
- TypeScript
module.exports = {
// ...
upload: {
config: {
sizeLimit: 250 * 1024 * 1024 // 256mb in bytes
}
}
};
export default {
// ...
upload: {
config: {
sizeLimit: 250 * 1024 * 1024 // 256mb in bytes
}
}
};
Upload request timeout
By default, the value of strapi.server.httpServer.requestTimeout
is set to 330 seconds. This includes uploads.
To make it possible for users with slow internet connection to upload large files, it might be required to increase this timeout limit. The recommended way to do it is by setting the http.serverOptions.requestTimeout
parameter in the config/servers
file.
An alternate method is to set the requestTimeout
value in the bootstrap
function that runs before Strapi gets started. This is useful in cases where it needs to change programmatically—for example, to temporarily disable and re-enable it:
- JavaScript
- TypeScript
module.exports = {
//...
bootstrap({ strapi }) {
// Set the requestTimeout to 1,800,000 milliseconds (30 minutes):
strapi.server.httpServer.requestTimeout = 30 * 60 * 1000;
},
};
export default {
//...
bootstrap({ strapi }) {
// Set the requestTimeout to 1,800,000 milliseconds (30 minutes):
strapi.server.httpServer.requestTimeout = 30 * 60 * 1000;
},
};
Responsive Images
When the Responsive friendly upload
admin panel setting is enabled, the plugin will generate the following responsive image sizes:
Name | Largest dimension |
---|---|
large | 1000px |
medium | 750px |
small | 500px |
These sizes can be overridden in /config/plugins
:
- JavaScript
- TypeScript
module.exports = ({ env }) => ({
upload: {
config: {
breakpoints: {
xlarge: 1920,
large: 1000,
medium: 750,
small: 500,
xsmall: 64
},
},
},
});
export default ({ env }) => ({
upload: {
config: {
breakpoints: {
xlarge: 1920,
large: 1000,
medium: 750,
small: 500,
xsmall: 64
},
},
},
});
Breakpoint changes will only apply to new images, existing images will not be resized or have new sizes generated.
Usage
The Media Library displays all assets uploaded in the application, either via the Media Library itself or via the Content Manager when managing a media field. Assets uploaded to the Media Library can be inserted into content-types using the Content Manager.
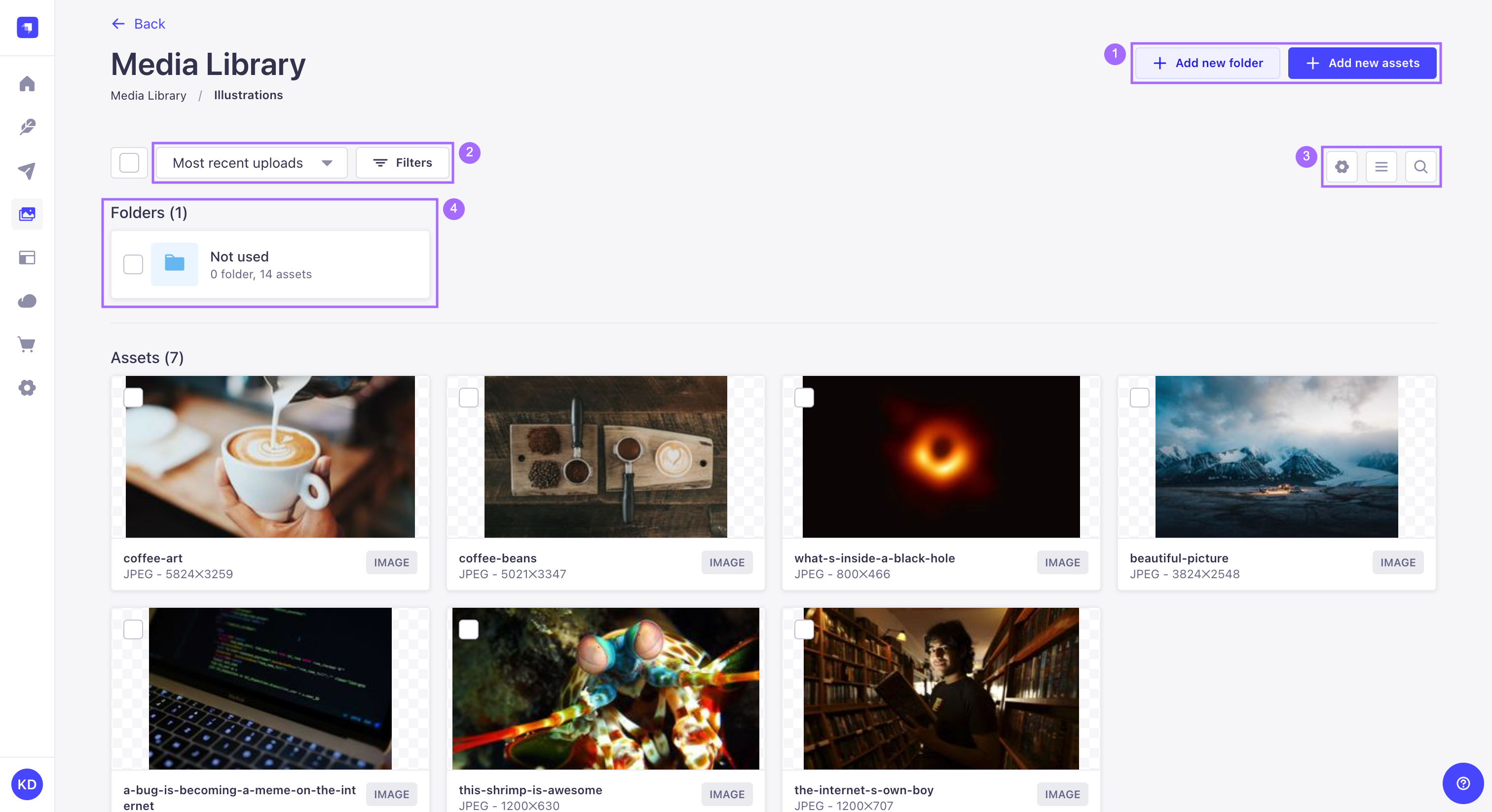
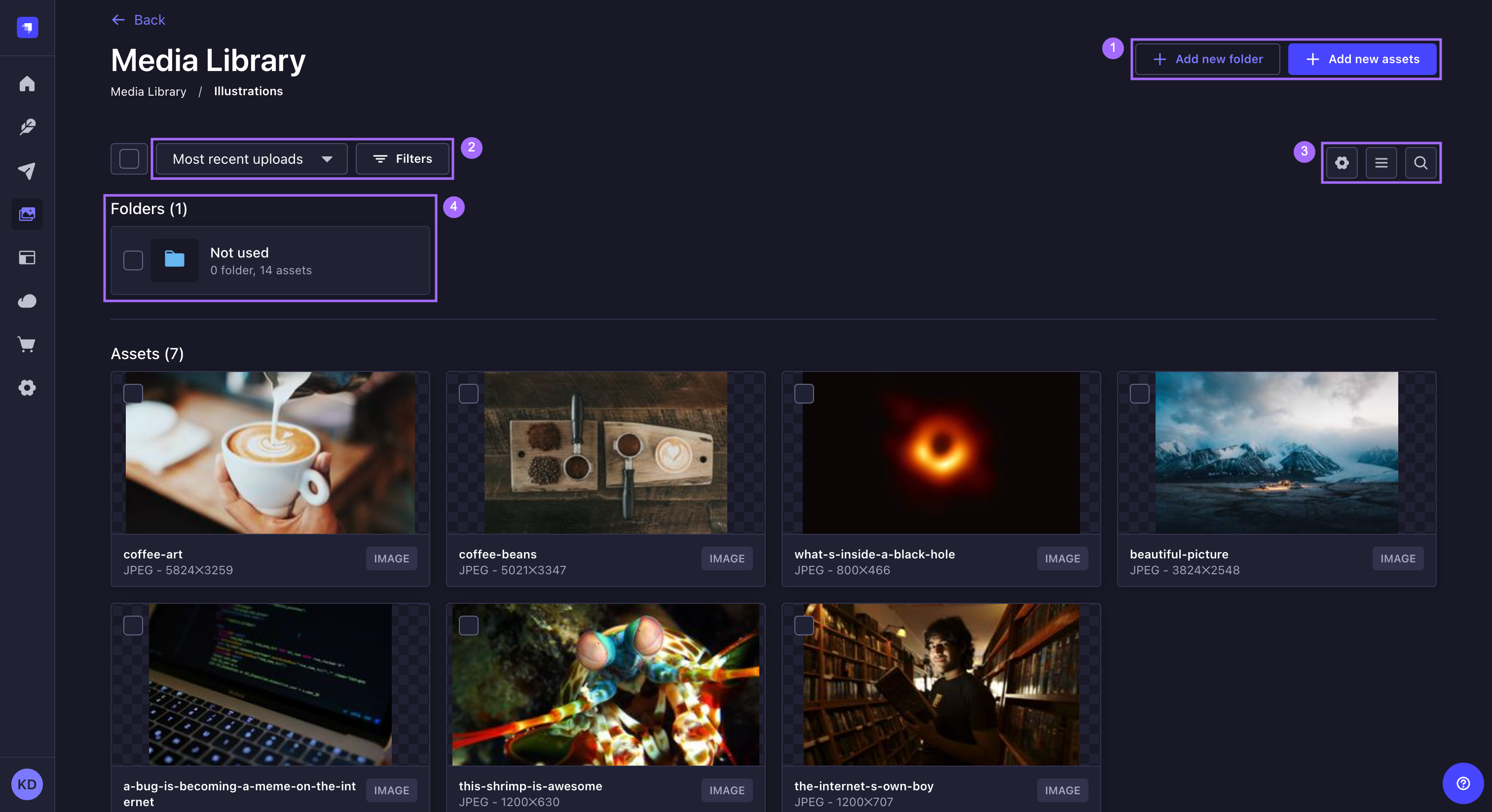
From the Media Library, it is possible to:
- upload a new asset (see adding assets) or create a new folder (see organizing assets with folders) 1,
- sort the assets and folders or set filters 2 to find assets and folders more easily,
- toggle between the list view
and the grid view
to display assets, access settings
to configure the view, and make a textual search
3 to find a specific asset or folder,
- and view, navigate through, and manage folders 4.
Click the search icon on the right side of the user interface to use a text search and find one of your assets or folders more quickly!
Filtering assets
Right above the list of folders and assets, on the left side of the interface, a Filters button is displayed. It allows setting one or more condition-based filters, which add to one another (i.e. if you set several conditions, only the assets that match all the conditions will be displayed).
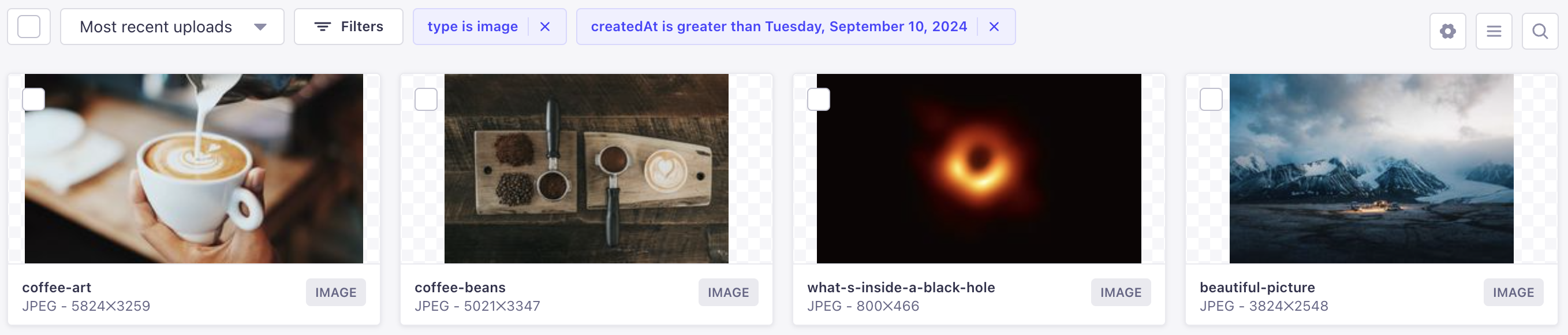
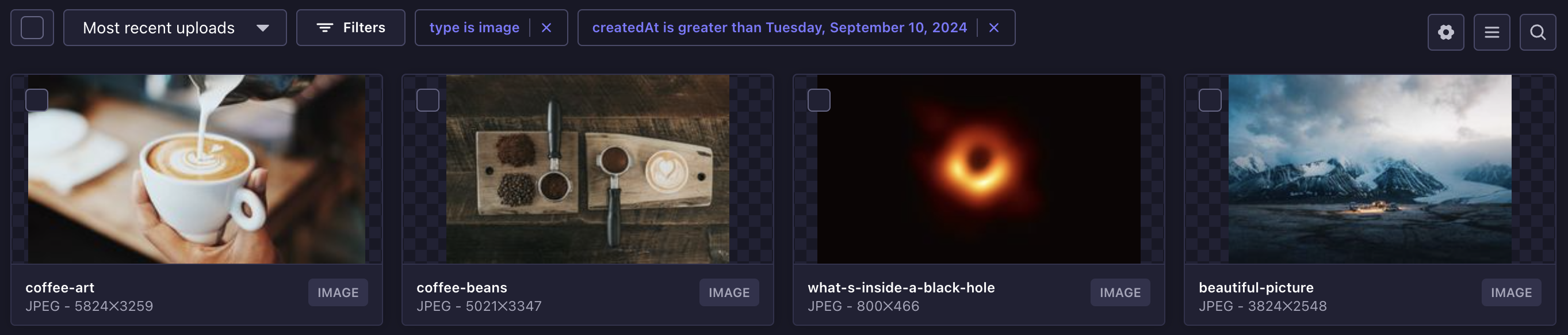
To set a new filter:
- Click on the
Filters button.
- Click on the 1st drop-down list to choose the field on which the condition will be applied.
- Click on the 2nd drop-down list to choose the type of condition to apply.
- For conditions based on the type of asset to filter, click on the 3rd drop-down list and choose a file type to include or exclude. For conditions based on date and time (i.e. createdAt or updatedAt fields), click on the left field to select a date and click on the right field to select a time.
- Click on the Add filter button.
When active, filters are displayed next to the Filters button. They can be removed by clicking on the delete icon
.
Sorting assets
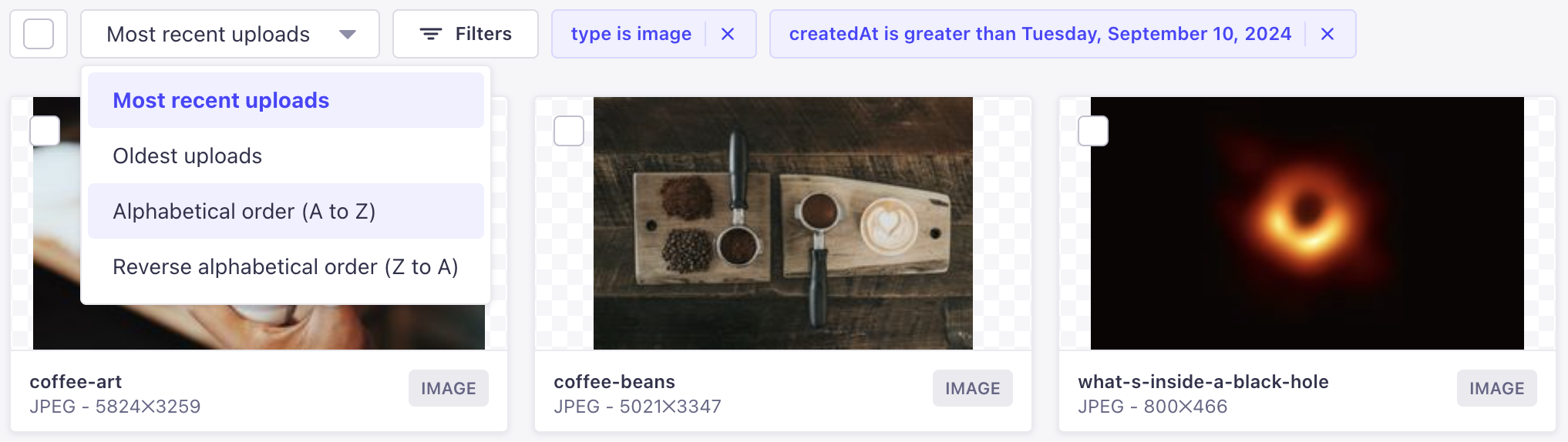
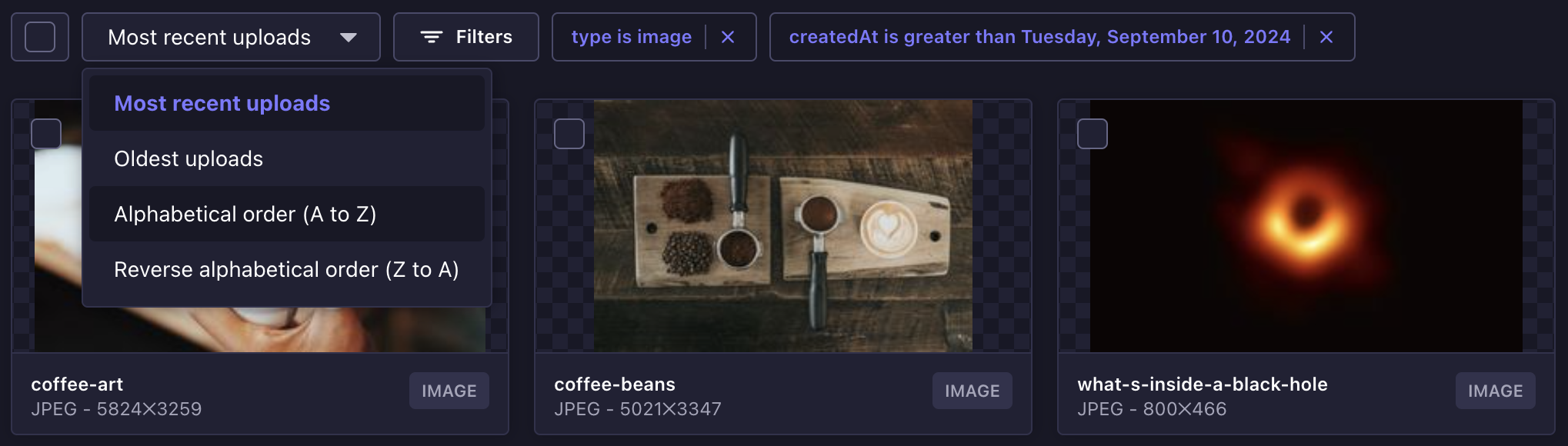
Just above the list of folders and assets and next to the Filters button, on the left side of the interface, a drop-down button is displayed. It allows to sort the assets by upload date, alphabetical order or date of update. Click on the drop-down button and select an option in the list to automatically display the sorted assets.
Configuring the view
Just above the list of folders and assets, on the right side of the interface, there is a group of 3 buttons. Click on to configure the default view for the Media library.
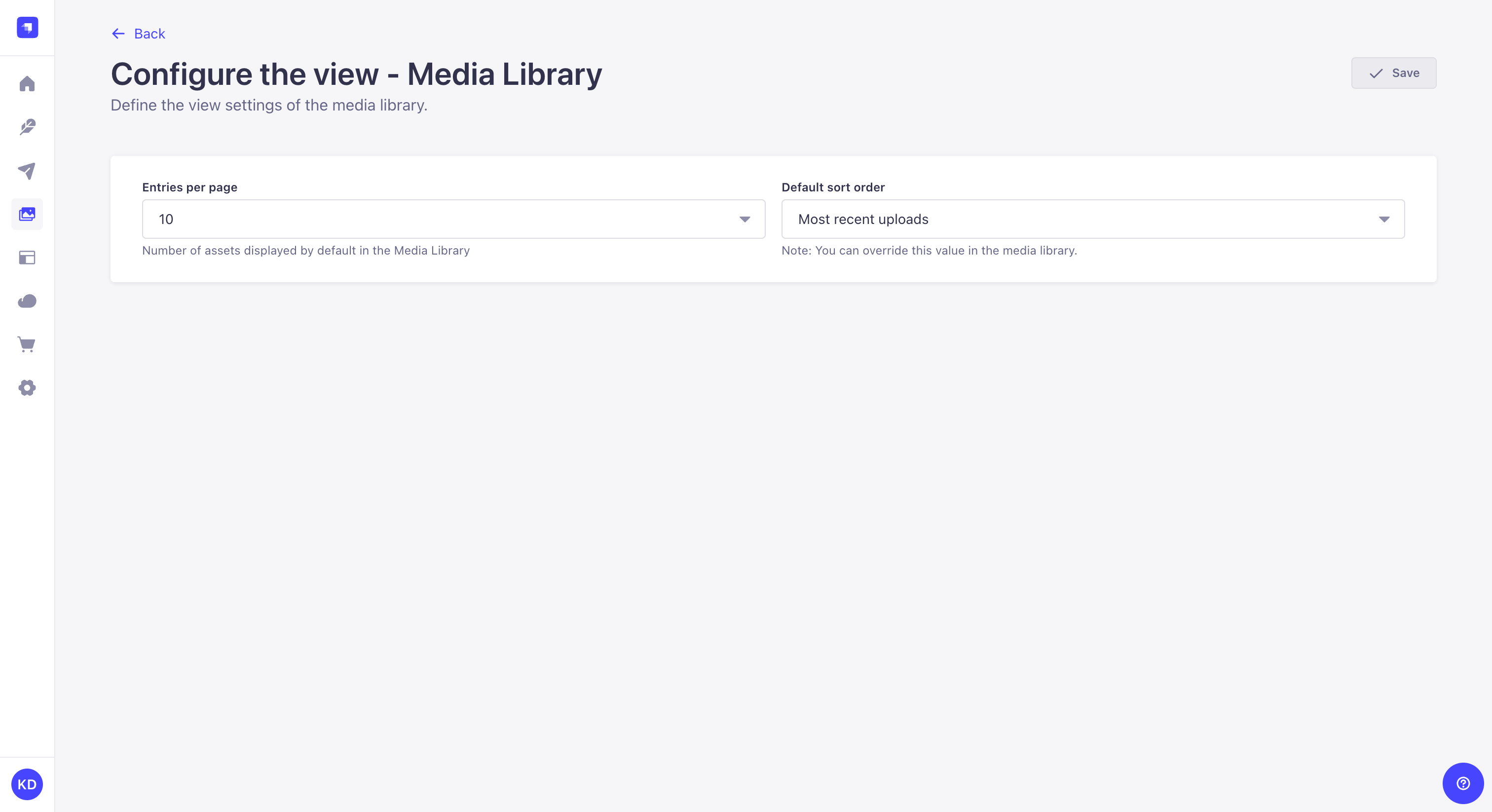
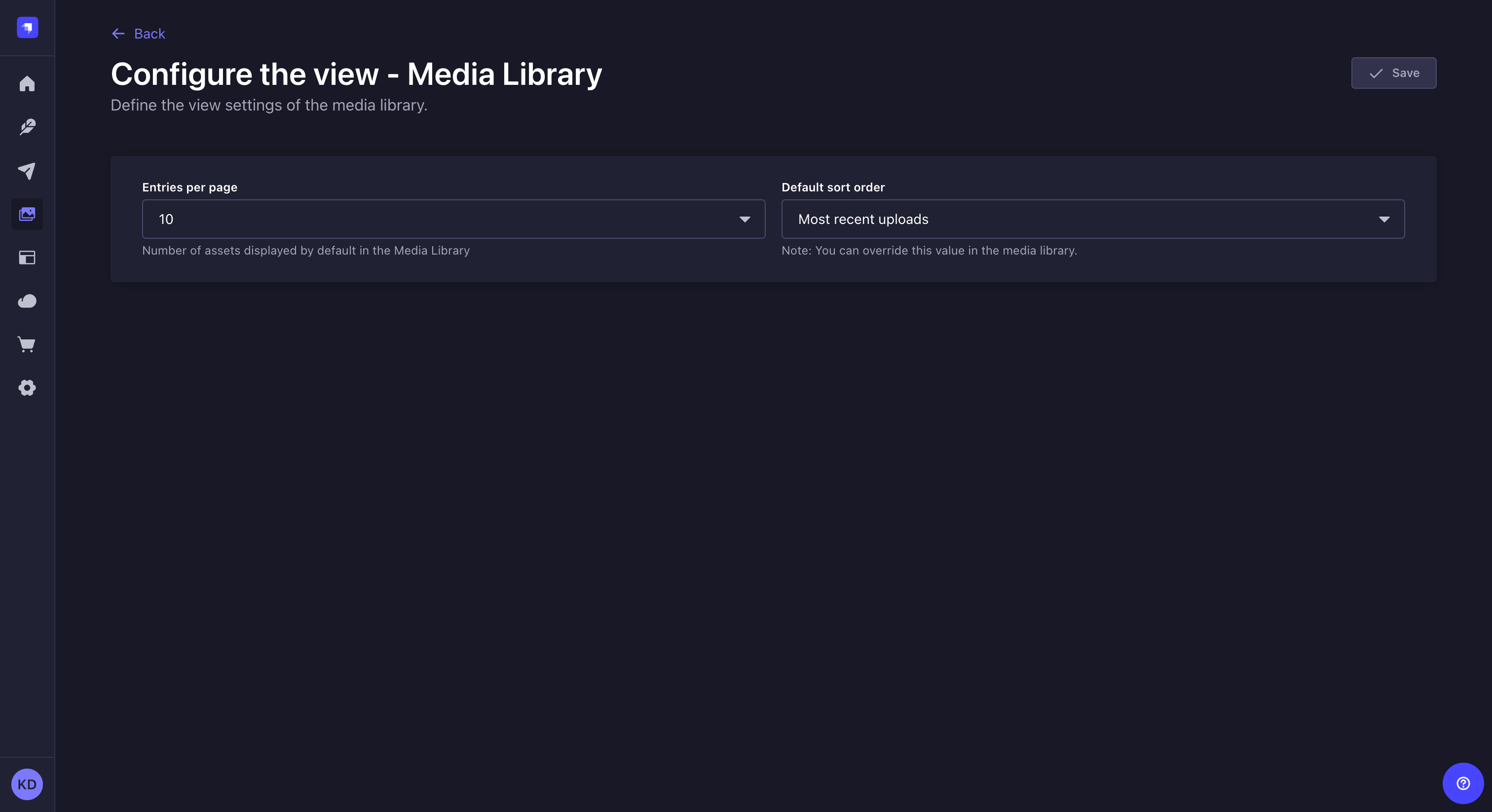
From there you can:
- Use the Entries per page dropdown to define the number of assets displayed by default
- Use the Default sort order dropdown the define the default order in which assets are displayed. This can be overriden when you sort assets in the Media Library.
Both settings are used as the defaults in the Media Library and in the Content Manager media upload modal. The settings saved here are global across the entire Strapi project for all users.
Adding assets
The Media Library displays all assets uploaded in the application, either via the Media Library or the Content Manager when managing a media field.
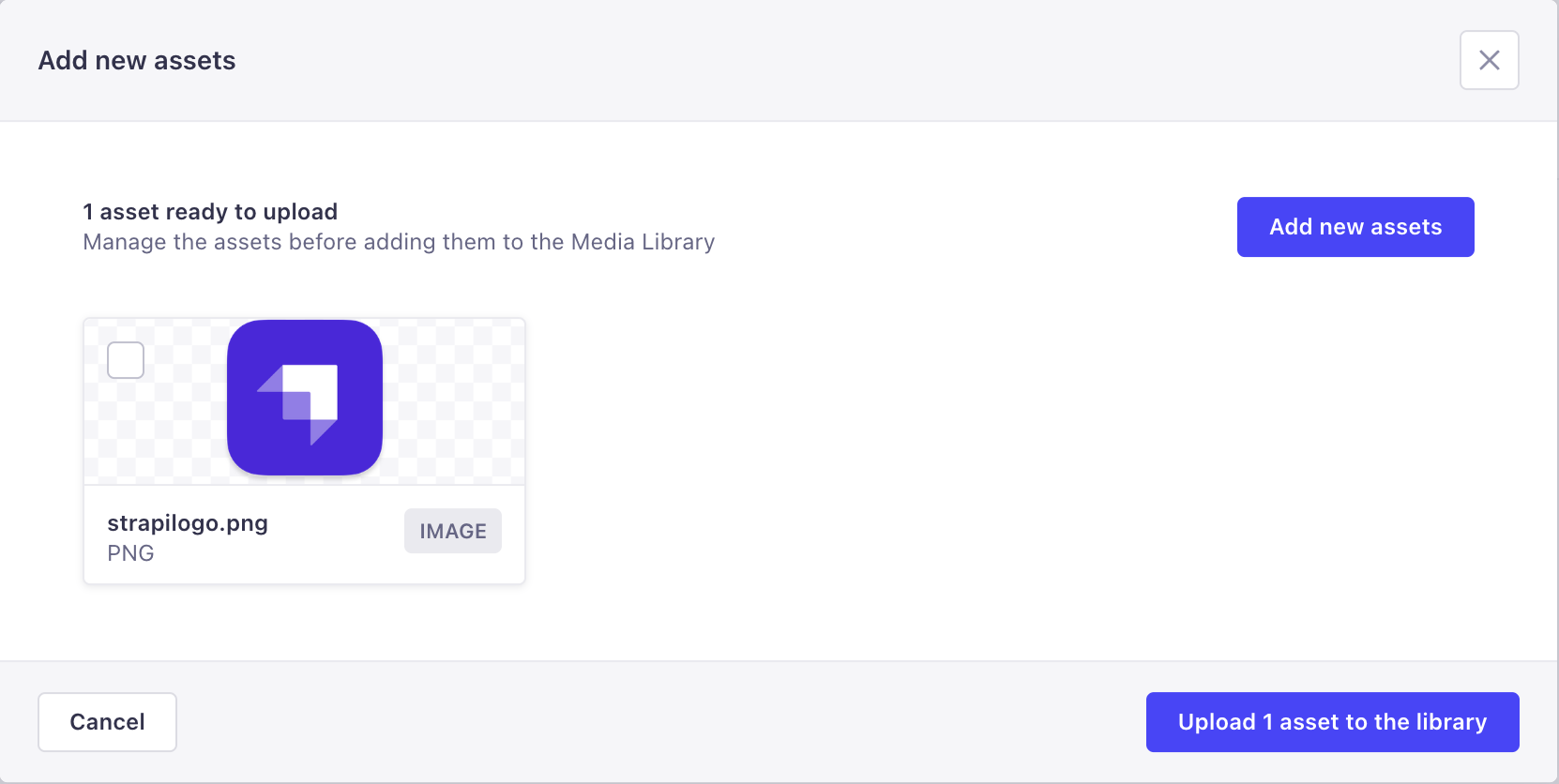
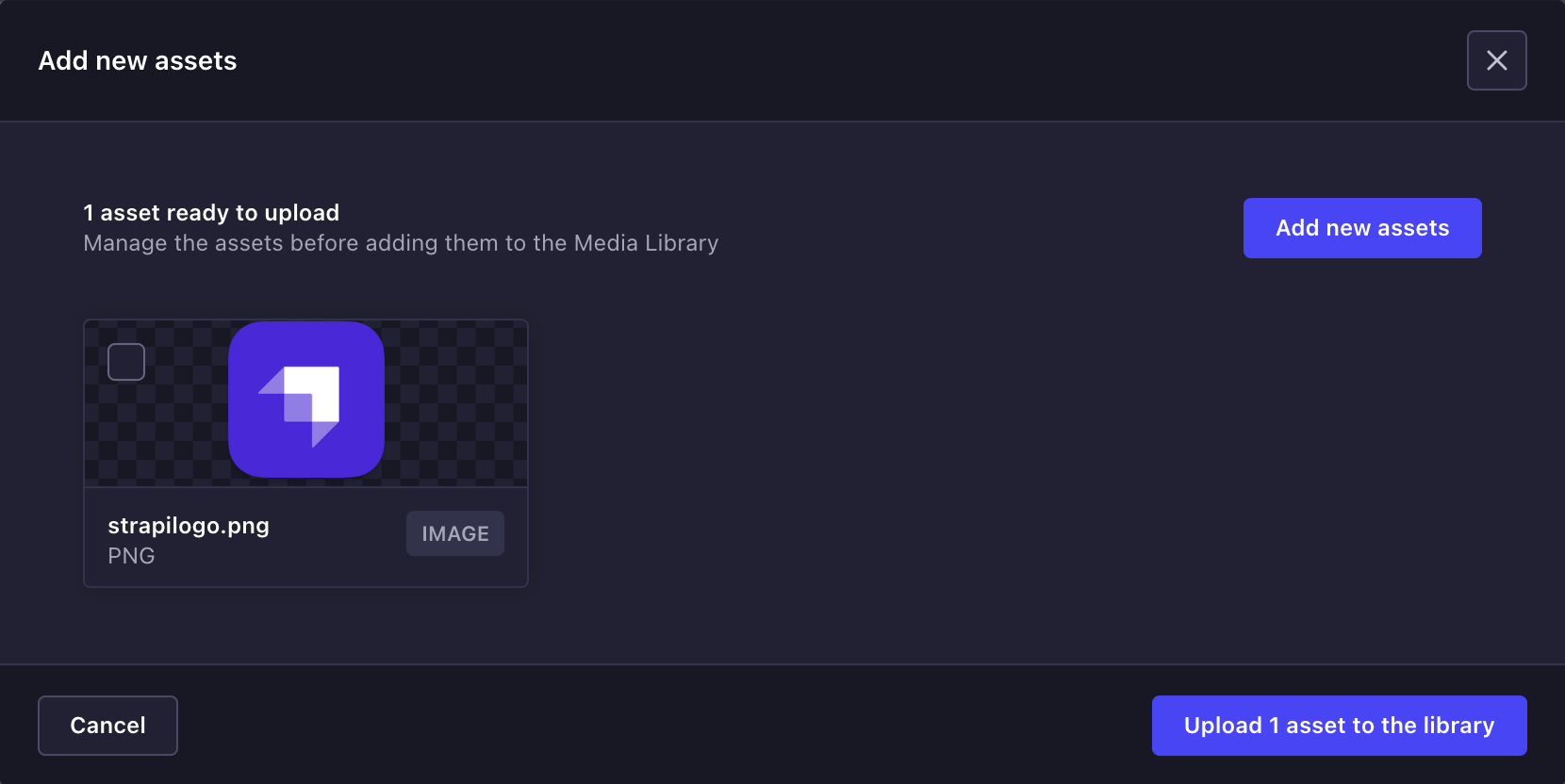
To add new assets to the media library:
- Click the Add new assets button in the upper right corner of the Media Library.
- Choose whether you want to upload the new asset from your computer or from an URL:
- from the computer, either drag & drop the asset directly or browse files on your system,
- from an URL, type or copy and paste an URL(s) in the URL field, making sure multiple URLs are separated by carriage returns, then click Next.
- (optional) Click the edit button
to view asset metadata and define a File name, Alternative text and a Caption for the asset (see editing and deleting assets).
- (optional) Add more assets by clicking Add new assets and going back to step 2.
- Click on Upload assets to the library.
A variety of media types and extensions are supported by the Media Library:
Media type | Supported extensions |
---|---|
Image | - JPEG - PNG - GIF - SVG - TIFF - ICO - DVU |
Video | - MPEG - MP4 - MOV (Quicktime) - WMV - AVI - FLV |
Audio | - MP3 - WAV - OGG |
File | - CSV - ZIP - XLS, XLSX - JSON |
Managing individual assets
The Media Library allows managing assets, which includes modifying assets' file details and location, downloading and copying the link of the assets file, and deleting assets. Image files can also be cropped. To manage an asset, click on its Edit button.
Editing assets
Clicking on the edit button of an asset opens up a "Details" window, with all the available options.
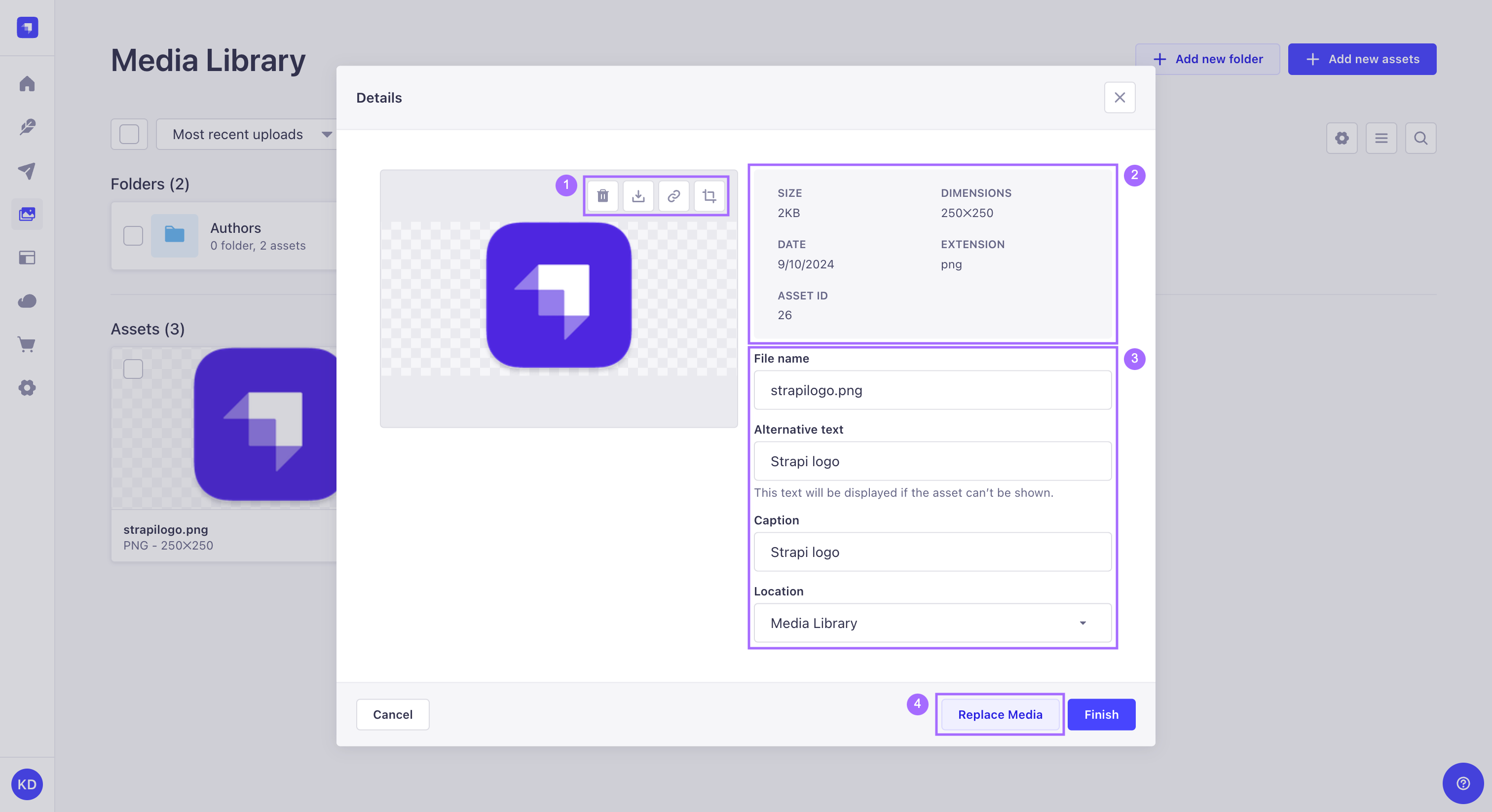
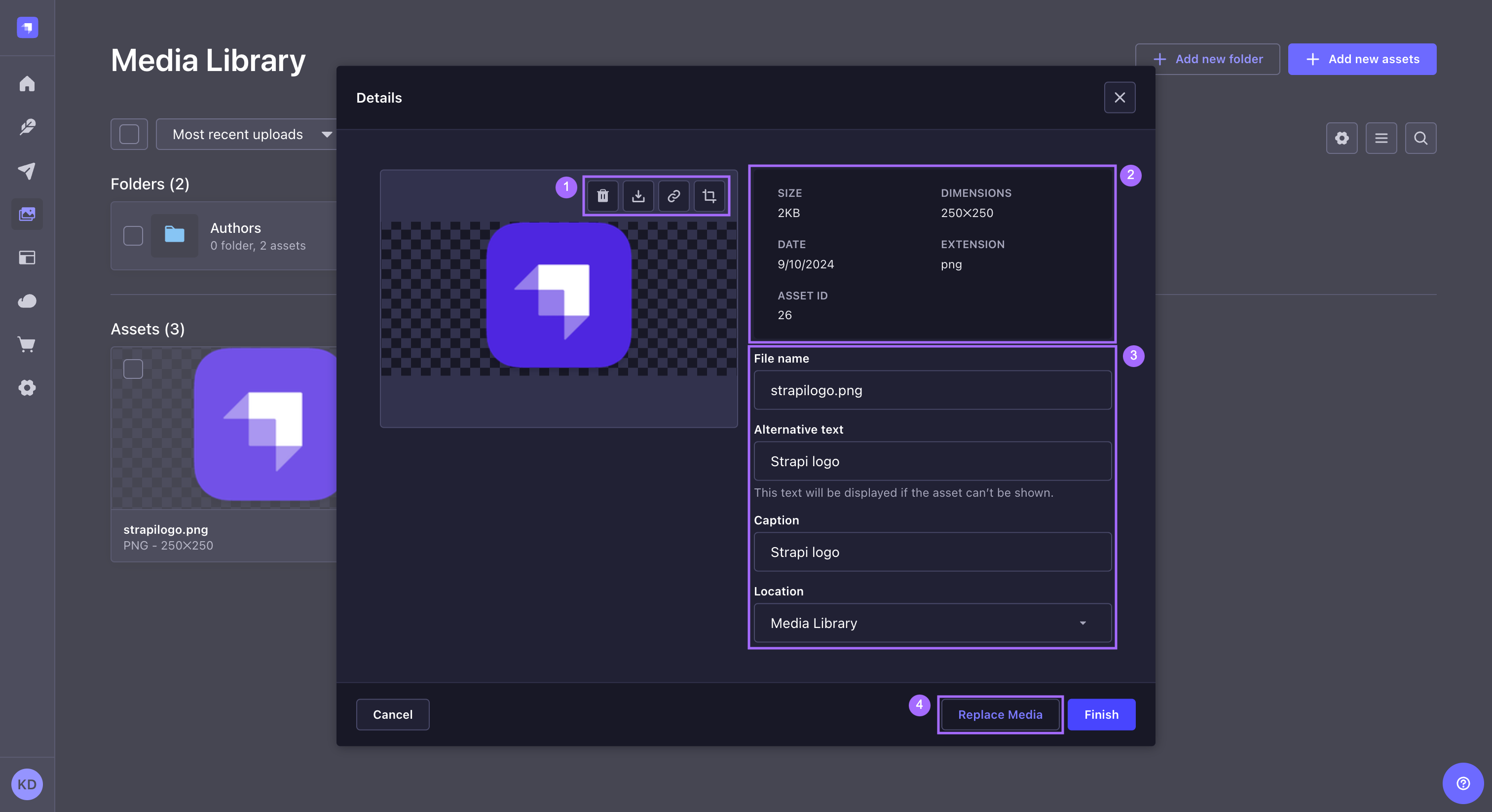
- On the left, above the preview of the asset, control buttons 1 allow performing various actions:
- click on the delete button
to delete the asset,
- click on the download button
to download the asset,
- click on the copy link button
to copy the asset's link to the clipboard,
- optionally, click on the crop button
to enter cropping mode for the image (see cropping images).
- click on the delete button
- On the right, meta data for the asset is displayed at the top of the window 2 and the fields below can be used to update the File name, Alternative text, Caption and Location (see organizing assets with folders) for the asset 3.
- At the bottom, the Replace Media button 4 can be used to replace the asset file but keep the existing content of the other editable fields, and the Finish button is used to confirm any updates to the fields.
Moving assets
An individual asset can be moved to a folder when editing its details.
To move an asset:
- Click on the edit
button for the asset to be moved.
- In the window that pops up, click the Location field and choose a different folder from the drop-down list.
- Click Save to confirm.
Assets can also be moved to other folders from the main view of the Media Library (see organizing assets with folders). This includes the ability to move several assets simultaneously.
Cropping images
Images can be cropped when editing the asset's details.
To crop an image:
- Click on the edit
button for the asset to be cropped.
- In the window that pops up, click the crop button
to enter cropping mode.
- Crop the image using handles in the corners to resize the frame. The frame can also be moved by drag & drop.
- Click the crop
button to validate the new dimensions, and choose either to crop the original asset or to duplicate & crop the asset (i.e. to create a copy with the new dimensions while keeping the original asset untouched). Alternatively, click the stop cropping
button to cancel and quit cropping mode.
- Click Finish to save changes to the file.
Deleting assets
An individual asset can be deleted when editing its details.
To delete an asset:
- Click on the edit
button for the asset to be deleted.
- In the window that pops up, click the delete button
in the control buttons bar above the asset's preview.
- Click Confirm.
Assets can also be deleted individually or in bulk from the main view of the Media Library. Select assets by clicking on their checkbox in the top left corner, then click the Delete icon at the top of the window, below the filters and sorting options.
Organizing assets with folders
Folders in the Media Library help you organize uploaded assets. Folders sit at the top of the Media Library view or are accessible from the Media field popup when using the Content Manager.
From the Media Library, it is possible to view the list of folders and browse a folder's content, create new folders, edit an existing folder, move assets to a folder, and delete a folder.
Folders follow the permission system of assets (see Users, Roles & Permissions). It is not yet possible to define specific permissions for a folder.
Browsing folders
By default, the Media Library displays folders and assets created at the root level. Clicking a folder navigates to this folder, and displays the following elements:
- the folder title and breadcrumbs to navigate to a parent folder 1
- the subfolders 2 the current folder contains
- all assets 3 from this folder
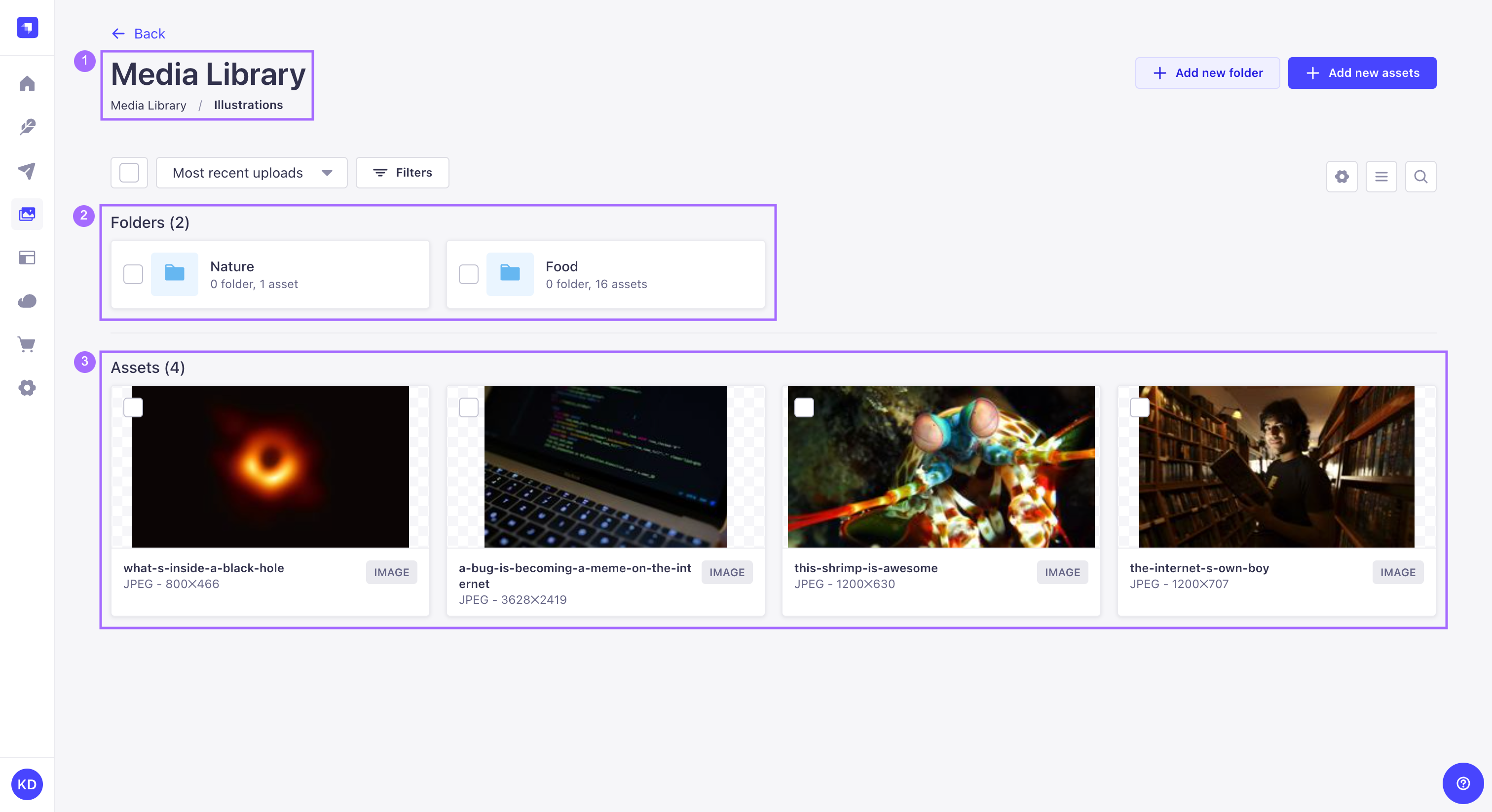
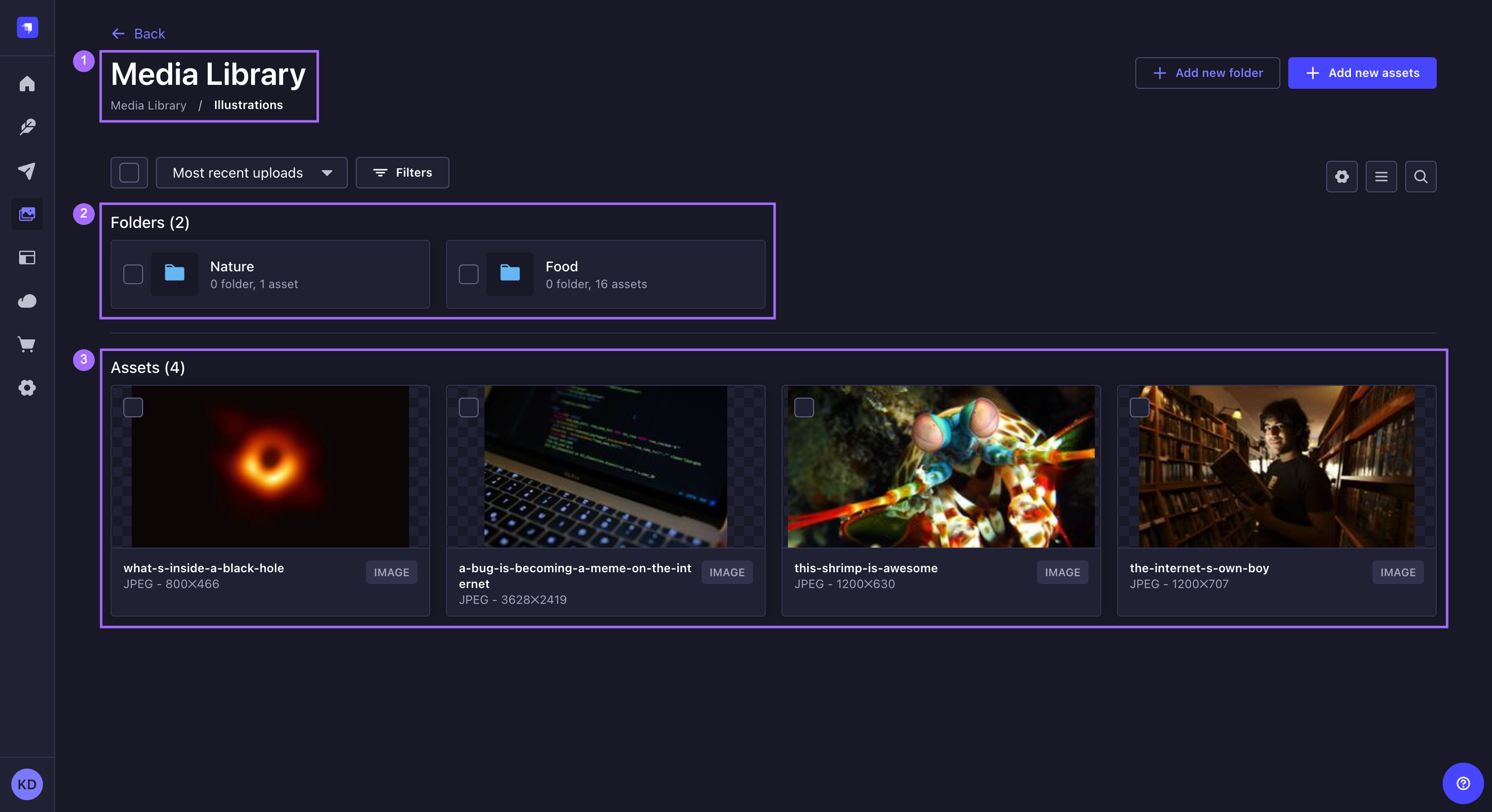
From this dedicated folder view, folders and assets can be managed, filtered, sorted and searched just like from the main Media Library (see introduction to Media Library).
To navigate back to the parent folder, one level up, use the Back button at the top of the interface.
The breadcrumb navigation can also be used to go back to a parent folder: click on a folder name to directly jump to it or click on the 3 dots /img.
and select a parent folder from the drop-down list.
Adding folders
To create a new folder in the Media Library:
- Click on Add new folder in the upper right of the Media Library interface.
- In the window that pops up, type a name for the new folder in the Name field.
- (optional) In the Location drop-down list, choose a location for the new folder. The default location is the active folder.
- Click Create.
There is no limit to how deep your folders hierarchy can go, but bear in mind it might take some effort to reach a deeply nested subfolder, as the Media Library currently has no visual hierarchy indication. Searching for files using the on the right side of the user interface might be a faster alternative to finding the asset you are looking for.
Moving assets to a folder
Assets and folders can be moved to another folder from the root view of the Media Library or from any view for a dedicated folder.
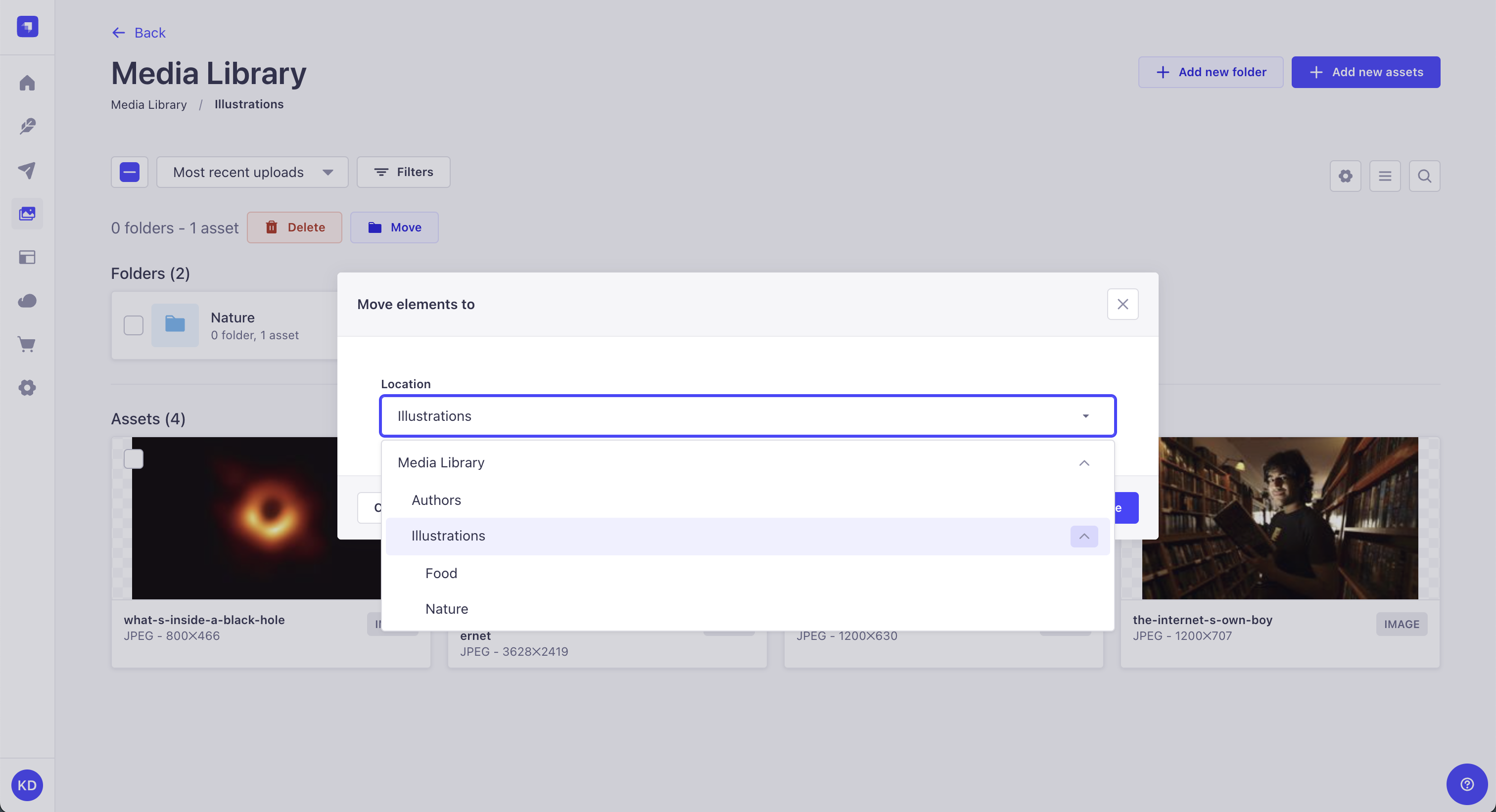
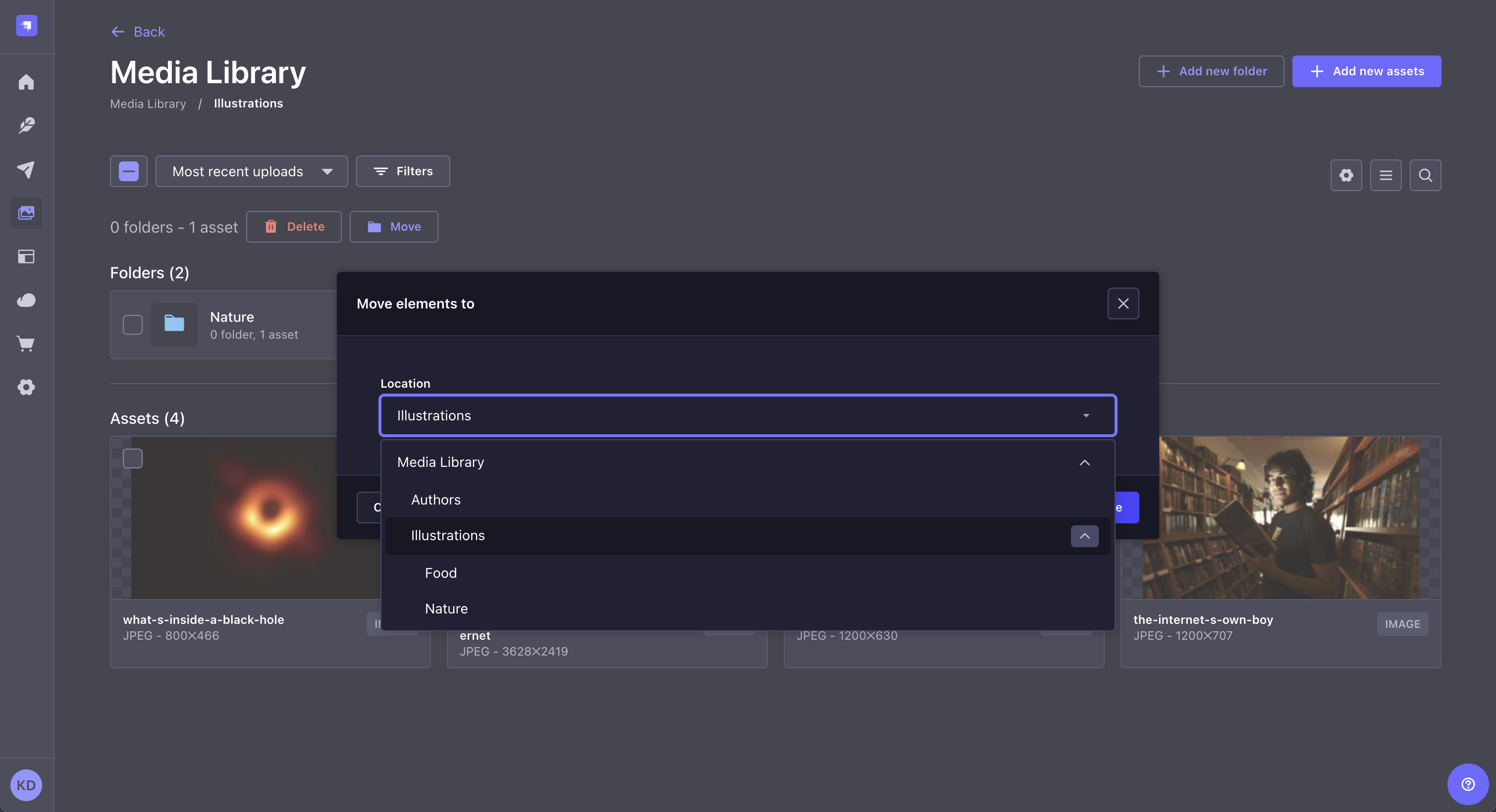
To bulk move assets and folders to another folder:
- Select assets and folder to be moved, by clicking the checkbox on the left of the folder name or clicking the asset itself.
- Click the
Move button at the top of the interface.
- In the Move elements to pop-up window, select the new folder from the Location drop-down list.
- Click Move.
An individual asset can also be moved to a folder when editing the asset.
Editing folders
Once created, a folder can be renamed, moved or deleted. To manage a single folder:
- In the Folders part of the Media library, hover the folder to be edited and click its edit button
.
- In the window that pops up, update the name and location with the Name field and Location drop-down list, respectively.
- Click Save.
Deleting folders
Deleting a folder can be done either from the list of folders of the Media Library, or when editing a single folder.
To delete a folder, from the Media Library:
- Click the checkbox on the left of the folder name. Multiple folders can be selected.
- Click the
Delete button above the Folders list.
- In the Confirmation dialog, click Confirm.
A single folder can also be deleted when editing it: hover the folder, click on its edit icon , and in the window that pops up, click the Delete folder button and confirm the deletion.
Usage with the REST API
The Media Library feature has some endpoints that can accessed through Strapi's REST API: